C# Magic, dispelling the illusion, illuminating the fun
Hi all!
Got a lot of positive comments after that talk, thank you all I'm so glad many of you enjoyed the whirlwind tour!
As promised I'm posting the slides up here (pdf) for people to look over and discuss, also available here as a GoogleDoc (fixed that closure thing and added a warning :D thx for the correction!)
If there are any glaring errors I missed I've still got the Google doc set up so I can make changes.
Otherwise if people have questions on usage, exact details, more on how Coroutines work in Unity (my model was *very* simplified, after all) then here would be a lovely place for us to chat and get those details sorted out for us to nerdgasm about. If I could, I'd suggest not posting "help me with my specific bug in my game" type questions here, you can always make another post... unless it's about how you fixed the bug with some interesting detail about X that everyone should be aware of, then hells yeah! It'd be nice to have a post where people can talk about general programming magic. But hey! what do I know, let's just nerd and see what happens :p
I'm not an expert, but I'll answer what I can. People with strong code-fu around here will probably be willing to share their knowledge as well :)
Love all 'y all ^_^
-rax
p.s: I'm working on a fuller model of how Unity runs its game-loop that I'll post up once I've done a bit or research, I think it might be a enlightening endevour.
Got a lot of positive comments after that talk, thank you all I'm so glad many of you enjoyed the whirlwind tour!
As promised I'm posting the slides up here (pdf) for people to look over and discuss, also available here as a GoogleDoc (fixed that closure thing and added a warning :D thx for the correction!)
If there are any glaring errors I missed I've still got the Google doc set up so I can make changes.
Otherwise if people have questions on usage, exact details, more on how Coroutines work in Unity (my model was *very* simplified, after all) then here would be a lovely place for us to chat and get those details sorted out for us to nerdgasm about. If I could, I'd suggest not posting "help me with my specific bug in my game" type questions here, you can always make another post... unless it's about how you fixed the bug with some interesting detail about X that everyone should be aware of, then hells yeah! It'd be nice to have a post where people can talk about general programming magic. But hey! what do I know, let's just nerd and see what happens :p
I'm not an expert, but I'll answer what I can. People with strong code-fu around here will probably be willing to share their knowledge as well :)
Love all 'y all ^_^
-rax
p.s: I'm working on a fuller model of how Unity runs its game-loop that I'll post up once I've done a bit or research, I think it might be a enlightening endevour.
pdf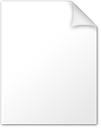
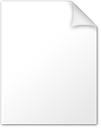
pdf
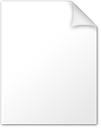
C# Magic.pdf
4M
Comments
[edit: will fix the colours asap :p can't right now]
So this was really useful to me. Thanks! :) Wish I could've seen it live.
[edit] Oh, I have struggled with Coroutines before. Especially if someone were to pause/unpause the game, or resume the game. It seems like quite a mission to find all the coroutines, save how long they've been running or what iteration they're on, stop them... and then start them again with whatever the correct timings are. I've mostly just ended up avoiding them. :(
I've seen some cool graphs of how Unity's game loop is constructed - so go out there and find them! :)
One thing that I would like to elaborate on is WHY to use coroutines, and to provide a small example. Let's say you have a complicated robot that does a million things, one of which is that it has a light on its head that switches between a bunch of different colours every once in a while. Without coroutines, you'd need a light switch timer/counter that decrements in Update and then switches colour, adding bloat to your already massive Update function and adding unnecessary variables EG:
Which could be replaced by a coroutine to do it for you:
My example is quite silly but I hope it demonstrates imo the biggest advantage of using coroutines: you can keep all the logic for an independent process in its own function AND you maintain the scope you had in that function. I.E. note how in the coroutine I don't have to check what the current state of the light is, as the function continues from the last point I yielded at.
(This is a shame, because I love the ideas behind it, and indeed use LINQ and enumerators for implementing algorithms all the time).
[edit] google provides http://wiki.unity3d.com/index.php?title=Singleton ^_^