[Elemental Shader Jam] Stormy Planet
Alrighty, so time to share some stuff, I will be doing quite a few things for this separately mostly as experimentation and learning (and these are things I have wanted to do for a long long time).
TexturedJitter
My goal with this one is to build a sort of "stormy planet" look, the first step works for a kind of Mars-ey/Saturn-ey storm planet look, though the next thing I will be doing is doing this with 2 textures to allow for a planet texture and then a cloud/storm texture. I initially wanted this to look like a flaming sun but I couldn't quite get that right, so on that I will head back to the drawing board.
The gif doesn't do it justice, but:
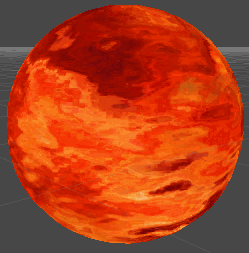
Code:
and texture used for people wanting to run it themselves:
TexturedJitter
My goal with this one is to build a sort of "stormy planet" look, the first step works for a kind of Mars-ey/Saturn-ey storm planet look, though the next thing I will be doing is doing this with 2 textures to allow for a planet texture and then a cloud/storm texture. I initially wanted this to look like a flaming sun but I couldn't quite get that right, so on that I will head back to the drawing board.
The gif doesn't do it justice, but:
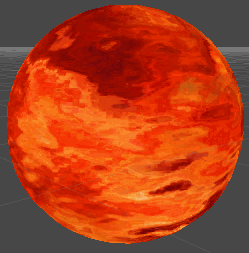
Code:
Shader "Custom/TexturedJitter" { Properties { _MainTex ("Main Texture", 2D) = "white" {} } SubShader { Pass { CGPROGRAM #include "UnityCG.cginc" sampler2D _MainTex; float4 _MainTex_ST; struct v2f { float4 pos : POSITION; half2 uv : TEXCOORD0; }; v2f vert (appdata_base v) { v2f o; o.pos = mul(UNITY_MATRIX_MVP, v.vertex); o.uv = TRANSFORM_TEX(v.texcoord, _MainTex); return o; } fixed4 frag (v2f i) : COLOR0 { fixed2 pos = tex2D(_MainTex, i.uv).xy; float pos_l = sqrt(pos.x * pos.x + pos.y + pos.y); pos = pos/pos_l; pos.x += cos(_Time.x); pos.y += sin(_Time.x * 3/2); fixed2 pos2 = (i.uv + pos)/(2 - sin(_Time.x)); // Look up those UVs in the texture fixed4 col = tex2D(_MainTex, pos2); return col; } #pragma vertex vert #pragma fragment frag ENDCG } } }
and texture used for people wanting to run it themselves:
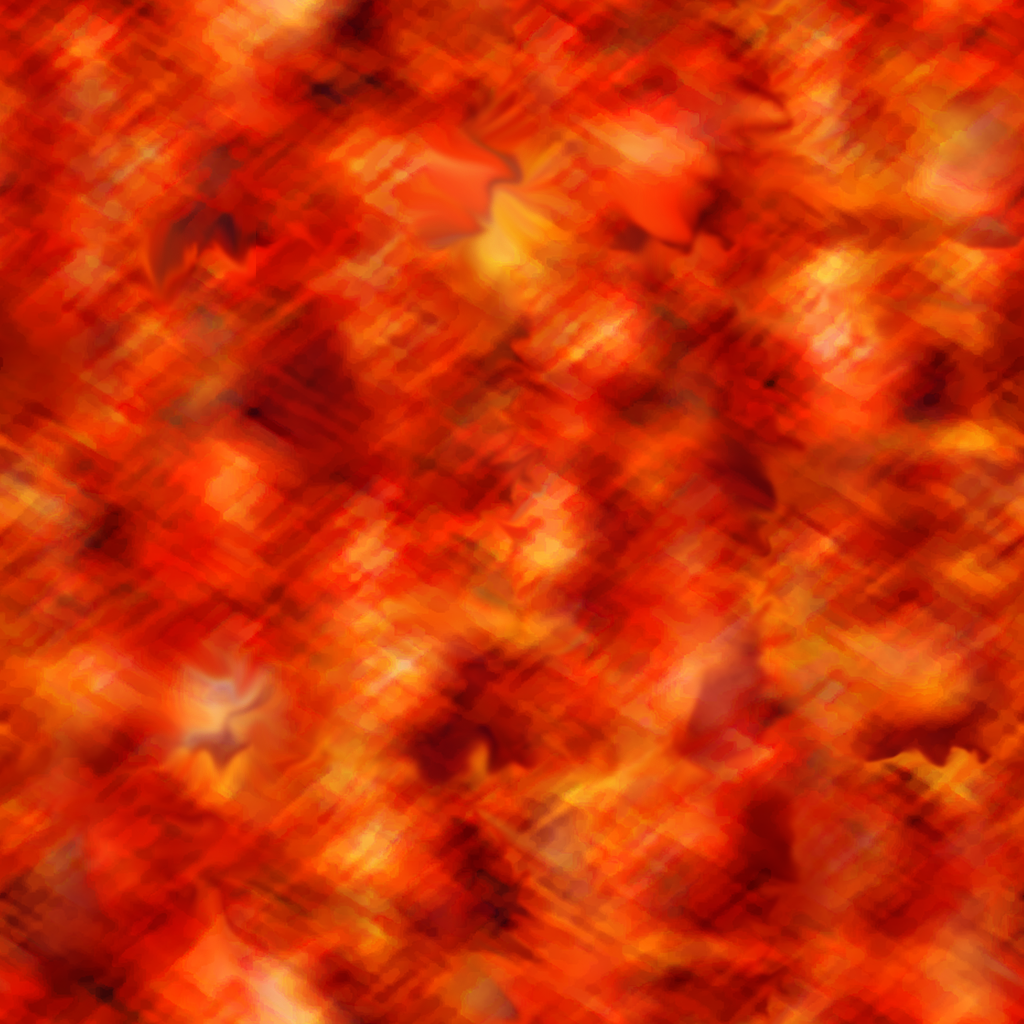
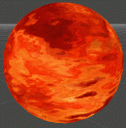
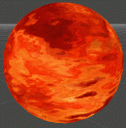
Example1.gif
249 x 253 - 2M
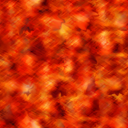
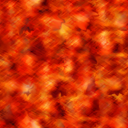
StarTexture.png
1024 x 1024 - 1M
Thanked by 1Fengol
Comments
This code is much better off written like so:
rsqrt is another intrinsic function that calculates recipricol square roots. Square roots are, generally speaking, pretty expensive operations, but there are tricks to calculating the inverse that are quite a bit faster. As a result, it's (usually) safe to assume the GPU instruction for rsqrt is going to be faster than calculating 1/sqrt, and it's good practice to use rsqrt where it makes sense. Alternately, to just never calculate the square root if you don't absolutely need to (like for normalising) :p
Also - arbitrary thread name change, Im assuming Im not allowed to post multiple shaders to a single thread?