General Math Questions
So, I have some mathematical questions I cannot get my tired brain around, and this is something I need for 2 separate projects, so here goes.
I am working on a space fighter of sorts and I'm trying to make a targeting system where you select what to attack and your ship manages the turning and firing for you, this has turned into something somewhat troublesome for me, and I cannot think of (or find) a good strategy to pull this off. Mathematically speaking I guess the problem is simple:
Im getting some really weird behaviours with the turn size changing arbitrarily and having random small loops (my target is sitting at 0,0,0 while I try work this out). I have tried lerping values with correct wrapping and I have tried Unity's Vector3.RotateTowards and I cannot get the right values for this to feel right.
What would be some good/interesting/potential solutions I could employ to make it feel like the ship is actually flying around?
I currently have random rotation and other things that aren't the desired effect, and my previous results (the fig-8) weren't promising:
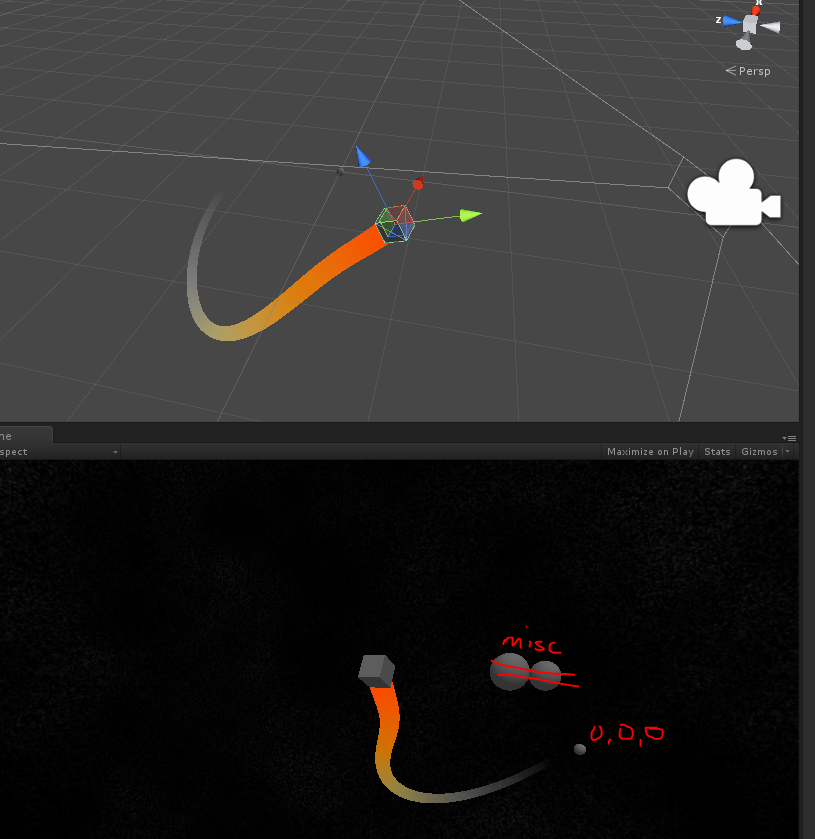
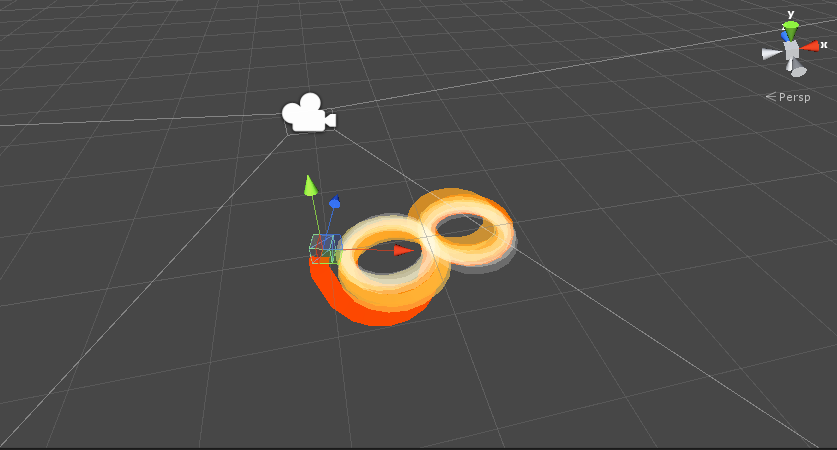
The code I am using is the following:
Which gives me the results in the first image, the ship randomly chooses a rotate direction and flies around erratically - however admittedly every now and then hitting the correct target.
In the image below you can see my targets and target direction seem to go towards the correct values (however this particular instance the ship got stuck flying past 0,0,0 in a straight line):
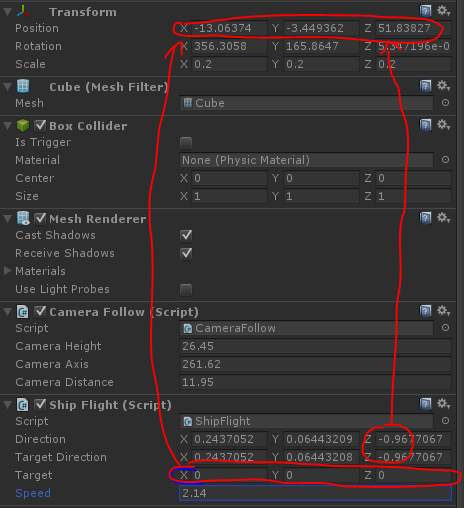
If there is some technique I can apply here that would be great, I am at a bit of a loss.
I am working on a space fighter of sorts and I'm trying to make a targeting system where you select what to attack and your ship manages the turning and firing for you, this has turned into something somewhat troublesome for me, and I cannot think of (or find) a good strategy to pull this off. Mathematically speaking I guess the problem is simple:
vector myPosition, myTarget, direction vector targetDirection = (myTarget - myPosition).normalized turn direction towards targetDirection
Im getting some really weird behaviours with the turn size changing arbitrarily and having random small loops (my target is sitting at 0,0,0 while I try work this out). I have tried lerping values with correct wrapping and I have tried Unity's Vector3.RotateTowards and I cannot get the right values for this to feel right.
What would be some good/interesting/potential solutions I could employ to make it feel like the ship is actually flying around?
I currently have random rotation and other things that aren't the desired effect, and my previous results (the fig-8) weren't promising:
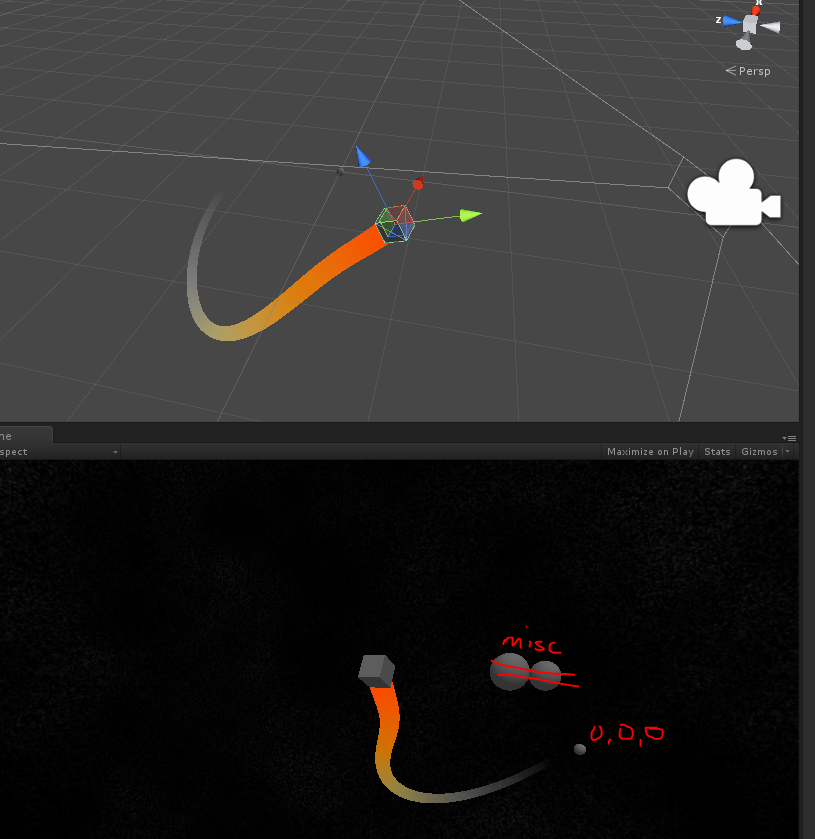
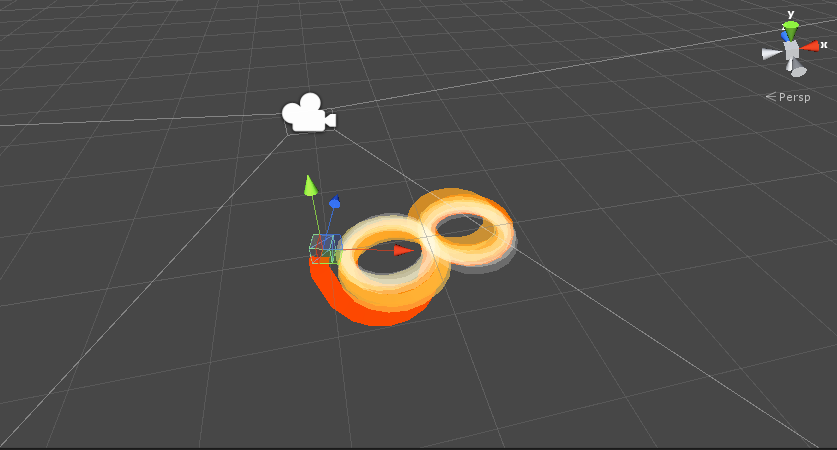
The code I am using is the following:
public class ShipFlight : MonoBehaviour { public Vector3 direction = new Vector3(); public Vector3 targetDirection = new Vector3(); public Vector3 target = new Vector3(); public float speed = 1; // Use this for initialization void Start () { } // Update is called once per frame void Update () { speed = Mathf.Clamp (speed, 1, 10); targetDirection = (target - gameObject.transform.position).normalized; direction = Vector3.RotateTowards (direction, targetDirection, Mathf.PI / 360, 0.1f).normalized; gameObject.transform.Translate (speed * direction * Time.deltaTime); gameObject.transform.LookAt (gameObject.transform.position + direction); } }
Which gives me the results in the first image, the ship randomly chooses a rotate direction and flies around erratically - however admittedly every now and then hitting the correct target.
In the image below you can see my targets and target direction seem to go towards the correct values (however this particular instance the ship got stuck flying past 0,0,0 in a straight line):
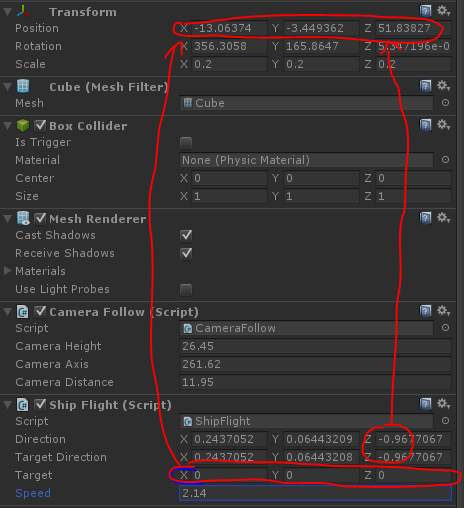
If there is some technique I can apply here that would be great, I am at a bit of a loss.
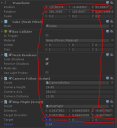
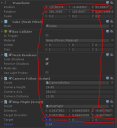
space.PNG
464 x 508 - 49K


space 2.PNG
815 x 839 - 327K
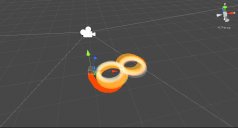
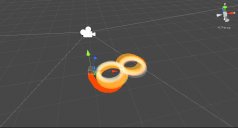
Around0zero.PNG
837 x 450 - 42K
Comments
In a test where the target was a original and the ship at (5,10,-20) I could happily play with turnSpeed values between 0.1 and 1
I needed my turrets to gradually track towards the player...
It kicks off when you get in range, and it shoots at you when you are even closer...
Same code is applied to different enemies (mines, bouncers and lazers)
It can be seen in action here...
http://makegamessa.com/discussion/1998/prototype-random-space-procedural-level-generation#Item_6
Here's the code... (maybe something useful in there)
See the Quaternion.RotateTowards bit... the Immediate Lookat also baffled me at first and looked unnatural.
Edit: Its a 2d Game so I only rotate on the Z... but could be adjusted.
Eye candy for results: