Share your old (and/or bad) code!
So! After discussing things last night with Gazza and Karuji and the likes we went into overdrive and discussed with vast quantities of nostalgia various projects we once worked on.
I am still working at getting stuff from old Game Maker projects, but in the mean time:
Project: "Galactic Jump" / Endless scrolling up-downy game.
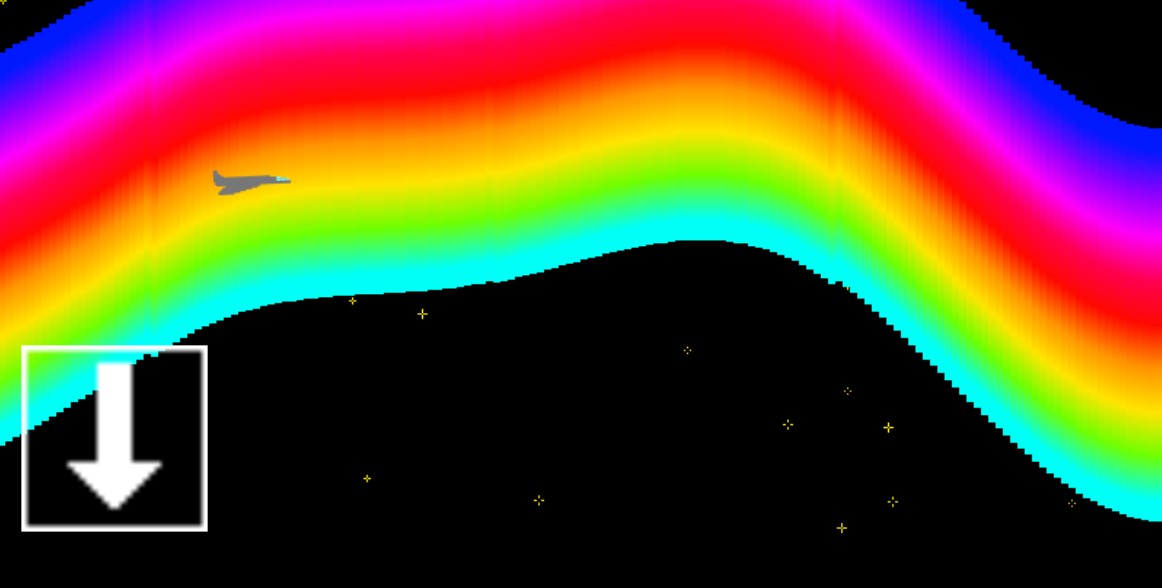
Built in XNA for Windows Phone 7 it was a competition entry to try and win golden circle tickets to see Kings of Leon. On the Windows Phone Store I ended up renaming it and changing it a few times, but here is my "rainbow generation code" which is the most interesting part of the code:
To describe what happens - I use cubic interpolation to work slowly from a certain width rainbow up and down (and connected to previous sections of rainbows). Not perfect, but could be useful for someone at some point.
Project: Fragmented Space / space shooter of sorts, boids implementation
This was a simple top down space shooter, the code behind it is very simple, but something I learned in the project was the concept of "boids". Essentially I built some simple flocking mechanics for my enemies:
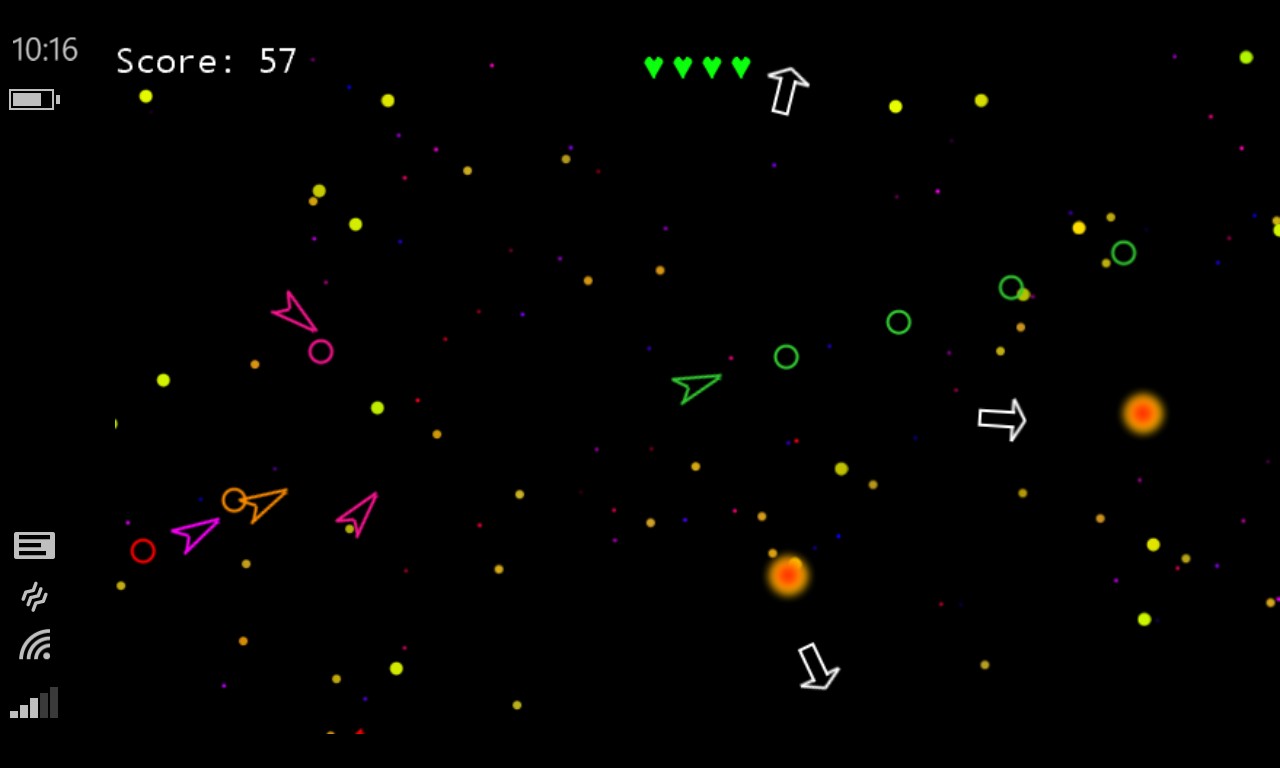
I still am going to go futher back and share code from older games (like my Competition 15 entry from way-back-when) if I can get the code. This idea stemmed from the fact that some of the games shown last night brought back thoughts of where I came from as a developer, and I wanted as an exercise to go over my old code and see what I thought.
I am still working at getting stuff from old Game Maker projects, but in the mean time:
Project: "Galactic Jump" / Endless scrolling up-downy game.
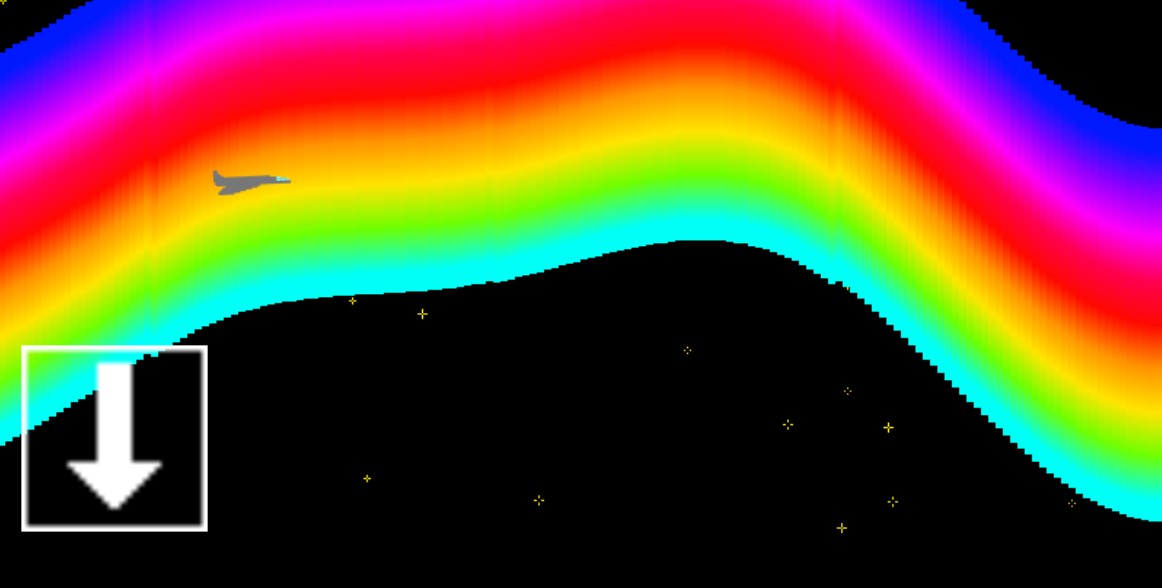
Built in XNA for Windows Phone 7 it was a competition entry to try and win golden circle tickets to see Kings of Leon. On the Windows Phone Store I ended up renaming it and changing it a few times, but here is my "rainbow generation code" which is the most interesting part of the code:
float CubicInterpolate(int y0, int y1, int y2, int y3, float mu) { int a0, a1, a2, a3; float mu2; mu2 = mu * mu; a0 = y3 - y2 - y0 + y1; a1 = y0 - y1 - a0; a2 = y2 - y0; a3 = y1; return (a0 * mu * mu2 + a1 * mu2 + a2 * mu + a3); } void SetUpInitialValues() { Score = 0; v2_plane_origin = new Vector2(t2d_plane.Width / 2, t2d_plane.Height / 2); v2_plane_position = new Vector2(128 + 16 + 32, 240); f_plane_movement = 0; f_plane_rotation = 0; SpacerSpeed = 5; CurrentOffset = MaxOffset; CurrentSpace = 470; OffSetCounter = 0; spacers = new List<SpacerPlacer>(); rainbows = new List<RainbowR>(); spacer_counter = 10000; Countdown = true; CountDownTimer = 5000; }
private bool DoSpacers() { bool crashed = false; for (int i = spacers.Count; i > 0; i--) { spacers[i - 1].placement.X -= SpacerSpeed; if (spacers[i - 1].placement.X < 0) spacers.RemoveAt(i - 1); else if (spacers[i - 1].placement.X > v2_plane_position.X - 16 && spacers[i - 1].placement.X < v2_plane_position.X + 16) { if ((v2_plane_position.Y > spacers[i - 1].placement.Y - 5 && v2_plane_position.Y < spacers[i - 1].placement.Y + 5) || (v2_plane_position.Y > spacers[i - 1].placement.Y + 480 - 5 && v2_plane_position.Y < spacers[i - 1].placement.Y + 480 + 5)) { crashed = true; } } } for (int i = rainbows.Count; i > 0; i--) { rainbows[i - 1].rect.X -= SpacerSpeed; if (rainbows[i - 1].rect.X < 0) rainbows.RemoveAt(i - 1); } if (spacer_counter <= 0) { if (CurrentSpace > MinSpace) CurrentSpace -= 25; if (SpacerSpeed < 10) SpacerSpeed += 1; spacer_counter = 10000; } if (!s_tunnel) { if (spc_rand.Next(250) > 248) { s_tunnel = true; tunnelcount = 5; } } else { tunnelcount -= 1; if (tunnelcount < 0) s_tunnel = false; } for (int i = 0; i < SpacerSpeed; i++) { OffSetCounter += spc_rand.Next(-2, 2); if (spc_rand.Next(250) > 240) { s_arbitrary = spc_rand.Next(45, 55); } CurrentOffset = (int)MathHelper.Clamp((float)(CurrentOffset + 3 * Math.Sin(OffSetCounter / s_arbitrary)), -MaxOffset, MaxOffset); CurrentSpace = (int)MathHelper.Clamp(CurrentSpace, MinSpace, 460); SpacerPlacer top, bottom; int usable_offset; if (spacers.Count > 3) { usable_offset = (int)(CubicInterpolate(spacers[spacers.Count - 3].Offset, spacers[spacers.Count - 1].Offset, CurrentOffset, CurrentOffset, 0.2f * i)); } else { usable_offset = CurrentOffset; } top = new SpacerPlacer(); top.placement.X = 850 - SpacerSpeed + i; top.placement.Y = usable_offset - 200 - (CurrentSpace / 2 - (s_tunnel == true ? 65 : 0)); top.Offset = CurrentOffset; spacers.Add(top); bottom = new SpacerPlacer(); bottom.placement.X = 850 - SpacerSpeed + i; bottom.placement.Y = (int)MathHelper.Clamp(usable_offset + (CurrentSpace / 2 - (s_tunnel == true ? 65 : 0)), 64, 460) + 240; bottom.Offset = CurrentOffset; spacers.Add(bottom); Rectangle rainbow = new Rectangle(850 - SpacerSpeed + i, top.placement.Y + 480, 3, (int)Math.Abs(top.placement.Y + 480 - bottom.placement.Y)); RainbowR r = new RainbowR(); r.rect = rainbow; rainbows.Add(r); } if (crashed) { Engine.ServiceOf<ScreenService>().PopScreen(); return true; } return false; }
To describe what happens - I use cubic interpolation to work slowly from a certain width rainbow up and down (and connected to previous sections of rainbows). Not perfect, but could be useful for someone at some point.
Project: Fragmented Space / space shooter of sorts, boids implementation
This was a simple top down space shooter, the code behind it is very simple, but something I learned in the project was the concept of "boids". Essentially I built some simple flocking mechanics for my enemies:
public class Enemy { public Vector2 Position; public float Direction; public Color Colour; public int FireRate = 1000; public int nextFire = 1000; public int Speed; private bool Evade = false; private int EvadeCount = 500; private int EvadeLength = 500; //BOIDS public Vector2 loc { get {return Position; } } public Vector2 vel = new Vector2(); public Vector2 acc = new Vector2(); float r; float maxSpeed; float maxForce; public Enemy(int x, int y, int speed, float mf) { Position = new Vector2(x, y); Speed = speed; r = 2.0f; maxSpeed = speed; maxForce = mf; } public void Run(List<Enemy> enemies) { Flock(enemies); //ChaseEvade(enemies); nextFire -= GameState.gameTime.ElapsedGameTime.Milliseconds; if (nextFire <= 0) { nextFire += FireRate; Bullet b = new Bullet(); b.Damage = 1; b.Direction = Direction; b.Speed = 2 * Speed; b.Colour = Colour; b.Position = new Vector2(Position.X, Position.Y); GameState.Bullets.Add(b); } Update(); } private void ChaseEvade(List<Enemy> enemies) { foreach (Enemy e in enemies) { Vector2 closest = new Vector2(); double d = 9999999; if (e.Colour != Colour) { double d2 = MHelper.Vector2Distance(Position, e.Position); if ((d2 < d) && (d2 > 25)) { d = d2; closest = e.Position; } } if (d < 50) { Evade = true; } double d3 = MHelper.Vector2Distance(e.Position, GameState.PlayerPosition); if ((d < d3) && (d3 > 75) && (!Evade)) { Direction = ChaseAndEvade.TurnToFace((float)Math.Atan2(closest.Y - Position.Y, closest.X - Position.X), Direction); } else if (Evade) { Direction = ChaseAndEvade.TurnToFace((float)Math.Atan2(GameState.PlayerPosition.Y - Position.Y, GameState.PlayerPosition.X - Position.X), -Direction); EvadeCount -= GameState.gameTime.ElapsedGameTime.Milliseconds; if (EvadeCount <= 0) { EvadeCount += EvadeLength; Evade = false; } } } } private void Flock(List<Enemy> enemies) { Vector2 sep = separate(enemies); Vector2 ali = align(enemies); Vector2 coh = cohese(enemies); Vector2 tar = target(); //weight forces sep *= 4.0f; ali *= 1.0f; coh *= 0.1f; tar *= 1.5f; acc = sep + ali + coh - tar; } private Vector2 target() { Vector2 chase = new Vector2(); float d = (float)MHelper.Vector2Distance(Position, GameState.PlayerPosition); if (d > 150) { chase = Position - GameState.PlayerPosition; chase.Normalize(); chase *= maxSpeed; if (chase.Length() > maxForce) { chase.Normalize(); chase *= maxForce; } } return chase; } private Vector2 cohese(List<Enemy> enemies) { float neighbourDistance = 1000f; Vector2 sum = new Vector2(); int count = 0; for (int i = 0; i < enemies.Count; i++) { Enemy other = enemies[i]; float d = (float)MHelper.Vector2Distance(loc,other.loc); if ((d < neighbourDistance) && (other.Colour == Colour) && (d > 0)) { sum += other.loc; count++; } } if (count > 0) { sum /= count; return steer(sum, false); } return sum; } private Vector2 align(List<Enemy> enemies) { float neighbourDist = 1000f; Vector2 steer = new Vector2(); int count = 0; for (int i = 0; i < enemies.Count; i++) { Enemy other = enemies[i]; float d = (float)MHelper.Vector2Distance(loc, other.loc); if ((d < neighbourDist) && (other.Colour == Colour) && (d > 0)) { steer += other.vel; count++; } } if (count > 0) steer /= count; if (steer.Length() > 0) { steer.Normalize(); steer *= maxSpeed; steer -= vel; if (steer.Length() > maxForce) { steer.Normalize(); steer *= maxForce; } } return steer; } private Vector2 separate(List<Enemy> enemies) { float desiredSeparation = 100f; Vector2 steer = new Vector2(); int count = 0; for (int i = 0; i < enemies.Count; i++) { Enemy other = enemies[i]; float d = (float)MHelper.Vector2Distance(loc, other.loc); if ((d < desiredSeparation) && (other.Colour == Colour) && (d > 0)) //colours flock together { Vector2 diff = loc - other.loc; diff.Normalize(); diff /= d; steer += diff; count++; } } if (count > 0) steer /= count; if (steer.Length() > 0) { steer.Normalize(); steer *= maxSpeed; steer /= vel; if (steer.Length() > maxForce) { steer.Normalize(); steer *= maxForce; } } return steer; } public void seek(Vector2 target) { acc += steer(target, false); } private void arrive(Vector2 target) { acc += steer(target, true); } private Vector2 steer(Vector2 target, bool slowDown) //steer towards a target { Vector2 steer = new Vector2(); Vector2 desired = target - loc; float d = desired.Length(); if (d > 0) { desired.Normalize(); if ((slowDown) && (d < 100.0f)) { desired *= maxSpeed * (d / 100.0f); } else { desired *= maxSpeed; } steer = desired - vel; if (steer.Length() > maxSpeed) { steer.Normalize(); steer *= maxSpeed; } } return steer; } private void Update() { vel += acc; if (vel.Length() > maxSpeed) { vel.Normalize(); vel *= maxSpeed; } Position += vel; acc *= 0; Direction = (float)Math.Atan2(vel.Y, vel.X); } }
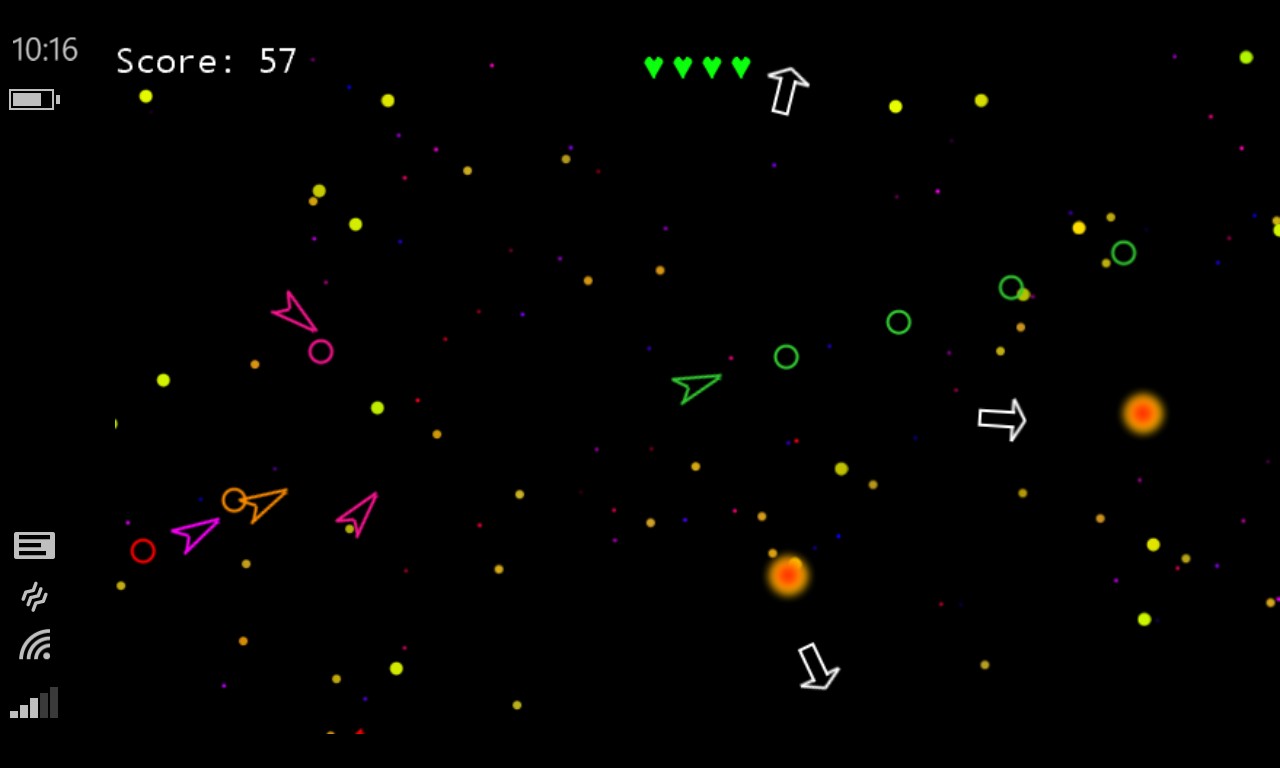
I still am going to go futher back and share code from older games (like my Competition 15 entry from way-back-when) if I can get the code. This idea stemmed from the fact that some of the games shown last night brought back thoughts of where I came from as a developer, and I wanted as an exercise to go over my old code and see what I thought.
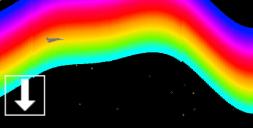
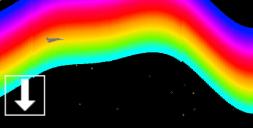
wp_ss_20140611_0001.jpg
1162 x 588 - 54K
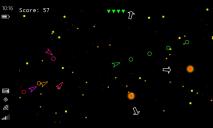
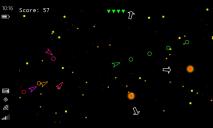
wp_ss_20140611_0002.jpg
1280 x 768 - 45K
Comments
.. brb
this was a bit interesting
not necc. bad code (except the keyDown[SDLK_ESCAPE] = false; wtf was I doing there?) just a shout out to my former self for making "YOU_LOSE_AND_YOU_CANT_WIN" a state in that game :D :D :D
Sprite someSprite = Resources.Load<Sprite>(string.Format("Textures/AniMAL/{0}", "elephant1"));
Eish! So each iteration it was fetching the same old sprites over and over and over and over and over.
Needless to say it stop crashing after this was moved to a non-looping method and cached... Doh :)
Don't drink and code!