Unity Particle System - starting velocity?
Helloooo
So while making Orbits for the past LD, I came across this problem that I've had for a long, long time but hadn't been able to figure out and had done tons of googling for.
I want to make a gameobject with a particle system, and I want that particle system to inherit velocity from the body from which it was made.
So, like a thing flies along a path, hits something. I want the resultant explosion/debris to go along with the same initial movement vector as the object was moving at.
So for example, these explosions don't have debris flying in the direction the object exploded from. I want to change these:
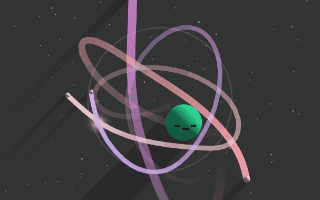
Now I hear you "yes there's a inherit velocity module on the particle system". Well yes, I looked into that, and I hadn't been able to get it to work. Instantiating a gameobject.
I could manually make gameobjects and hurl them in the vector, but that's more overhead. I want PARTICLES! How do I control particle emitters??
So while making Orbits for the past LD, I came across this problem that I've had for a long, long time but hadn't been able to figure out and had done tons of googling for.
I want to make a gameobject with a particle system, and I want that particle system to inherit velocity from the body from which it was made.
So, like a thing flies along a path, hits something. I want the resultant explosion/debris to go along with the same initial movement vector as the object was moving at.
So for example, these explosions don't have debris flying in the direction the object exploded from. I want to change these:
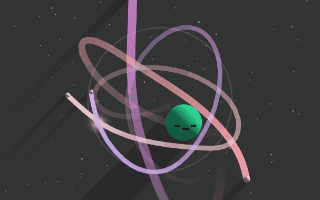
Now I hear you "yes there's a inherit velocity module on the particle system". Well yes, I looked into that, and I hadn't been able to get it to work. Instantiating a gameobject.
I could manually make gameobjects and hurl them in the vector, but that's more overhead. I want PARTICLES! How do I control particle emitters??
Comments
As for spawning with a cone, yeah I thought about that, but that means if the velocity is not the same every time, the particles will go at the same speed. IE a slow projectile will explode with the vigour of a bullet train crash :/
You'd then want to leave the particle system on the gameobject, but turn the emission module off, and when you want it to emit, you turn it on instead of spawning one in.
https://docs.unity3d.com/ScriptReference/ParticleSystem-emission.html
I understand that approach and it feels cumbersome, and also I have to attach a variety of particle emitters to the object, instead of pulling what I need at will.
So what I'm trying to find out is - could I access the velocity at start or velocity over time module of a particle system, so I can instantiate or activate (if pooling), and then pass the velocity data into it? Or is that too damn hard? :/
If you're trying to access the velocity over time module, you can via this: https://docs.unity3d.com/ScriptReference/ParticleSystem-velocityOverLifetime.html
Doing either of those seems just as much work (if not more) than just enabling the emission module though.
The Unity particle editor's pretty rudimentary, and they just expose lots of it in the scripting to try make up for it.
Just make sure you're following the examples in the docs. "Particle system modules do not need to be reassigned back to the system; they are interfaces and not independent objects" -- which is different to pretty much everything else in Unity... <_<
Edit. Maybe this is a bit more detailed of how I would do it (untested as I am at work atm)
And what I've been doing is simply to tell the particlesystem to play() when it hits. Does the particlesystem not know its velocity before it's playing? I tried changing the emission to be play on awake and added a constant looping particle, and those behave correctly (ie followed the velocity of the body) but if I turn play off and on when it needs to activate it doesn't work.
I tried following your emissions on/off method and unity tells me "UnityEngine.ParticleSystemEmissionType' is obsolete:"
I thought it might still work and tried it but it seems that the emissions module example in the documentation (https://docs.unity3d.com/ScriptReference/ParticleSystem-emission.html) is inserting bursts at runtime, I tried to insert a burst at 0f seconds but it didn't do anything.............
I can't believe how hard this is -_____-
I tested this and it worked.
This part spawns the particle system and sets the startingSpeed variable before its spawned
This part sets the starting speed of the system in the starting method.