Pathfinding
As promised, an explanation of how I do path finding.
The important thing to me is not where my character is coming from, but where he wants to go. As such, I apply my path finding in reverse and then I re-use it for various things in my game. I can make my character flee to the safest possible area, I can alter his course in-flight without having to recalculate and I can use the same path finding route for each and every character on my map, so I only need to calculate it once per Update() call or if nothing has changed I don't have to update it at all. I can pre-optimize certain paths and I could also apply preferential treatment or benefits to some paths and penalise others.
For this example the basic rules for movement limit you to horizontal and vertical moves. So no diagonal moves allowed.
It is pretty simple. Imagine this game map, which has land with a few water obstacles.
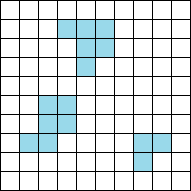
Next add your hero (represented by a red square) and 4 NPC's trying to get to him (represented in green squares)
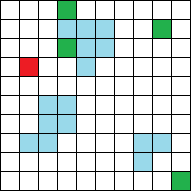
You need them to each have a unique path to reach him, right?
So I go back a step at the point, and I remove my NPC's from the equation. After all, I don't care where my NPC's are, I simply want them to be able to reach my hero. What is important to my NPC's is the terrain they have to cross, nothing more. So I go to the destination and I mark each passable tile based on it's distance to the hero.
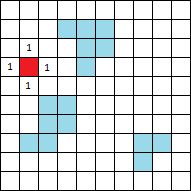
Next, I go to each tile next to the marked tiles and mark them based on their distance to the hero.
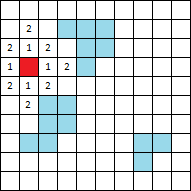
Again. repeat the process
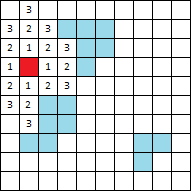
Until you have marked the entire range of tiles that are of interest to your or covers your entire map
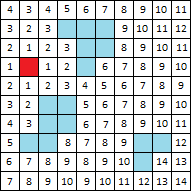
Now it's easy. Wherever your NPC is, he has to move to the next tile that has a smaller distance to the tile that he is currently standing on to move closer to the hero, or he has to do the opposite to move away from the hero.
The following figure shows how each of the NPC's can derive a unique path to the hero
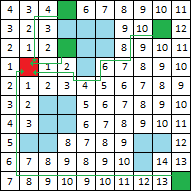
Based on this, I am able to do lots of small little 'world awareness' things in my game without actually having to do anything, it's embedded in my map. Calculating paths had just become blisteringly fast and as a result, I can now make my surroundings and tiles behave based on distance to the Hero which makes the world feel a little more alive. At least that is the possibility. I am still working on it, but hey, who knows :)
So the bottom line is, the world plays a vital part in path finding and using this kind of mechanism you can have your world give your character feedback on what to do when. Imagine him ducking instinctively when he walks by a tree with a low hanging branch for example. Or the wind howling become more evident as you approach the edge of a cliff.
I hope this wasn't a complete waste of your times, but how does this compare to what you guys use?
The important thing to me is not where my character is coming from, but where he wants to go. As such, I apply my path finding in reverse and then I re-use it for various things in my game. I can make my character flee to the safest possible area, I can alter his course in-flight without having to recalculate and I can use the same path finding route for each and every character on my map, so I only need to calculate it once per Update() call or if nothing has changed I don't have to update it at all. I can pre-optimize certain paths and I could also apply preferential treatment or benefits to some paths and penalise others.
For this example the basic rules for movement limit you to horizontal and vertical moves. So no diagonal moves allowed.
It is pretty simple. Imagine this game map, which has land with a few water obstacles.
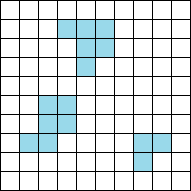
Next add your hero (represented by a red square) and 4 NPC's trying to get to him (represented in green squares)
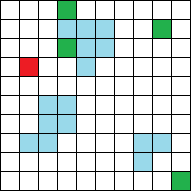
You need them to each have a unique path to reach him, right?
So I go back a step at the point, and I remove my NPC's from the equation. After all, I don't care where my NPC's are, I simply want them to be able to reach my hero. What is important to my NPC's is the terrain they have to cross, nothing more. So I go to the destination and I mark each passable tile based on it's distance to the hero.
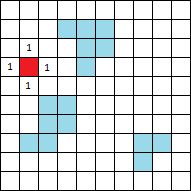
Next, I go to each tile next to the marked tiles and mark them based on their distance to the hero.
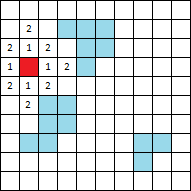
Again. repeat the process
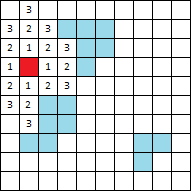
Until you have marked the entire range of tiles that are of interest to your or covers your entire map
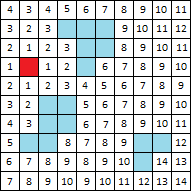
Now it's easy. Wherever your NPC is, he has to move to the next tile that has a smaller distance to the tile that he is currently standing on to move closer to the hero, or he has to do the opposite to move away from the hero.
The following figure shows how each of the NPC's can derive a unique path to the hero
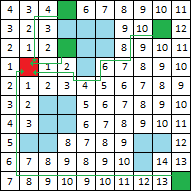
Based on this, I am able to do lots of small little 'world awareness' things in my game without actually having to do anything, it's embedded in my map. Calculating paths had just become blisteringly fast and as a result, I can now make my surroundings and tiles behave based on distance to the Hero which makes the world feel a little more alive. At least that is the possibility. I am still working on it, but hey, who knows :)
So the bottom line is, the world plays a vital part in path finding and using this kind of mechanism you can have your world give your character feedback on what to do when. Imagine him ducking instinctively when he walks by a tree with a low hanging branch for example. Or the wind howling become more evident as you approach the edge of a cliff.
I hope this wasn't a complete waste of your times, but how does this compare to what you guys use?
Thanked by 1Bensonance
Comments
It's a solid approach to 2D pathfinding, I think Desktop Dungeons uses something very similar.
So in A* if you have 10 characters moving, that's 10 searches. I have one path and each character that wants to move can move. He hardly has to think about it...
Not sure if that explains it a little better?
For me 2D is it, I have no aspirations for 3D as it makes me motion sick (for days!). So while there might very well be fantastic techniques for 3D the chances of me actually using them are slim, I suppose, without implying that I'm limiting possibilities here. So that's a bit of a limit to my exposure there. I haven't used it extensively yet, so I suppose that there are pitfalls around the corner at some point. What I like about it is that I can group things together in my mind and have some common logic for common things in Zombie Apocalypse. Like all things, I don't see it as the silver bullet, but as one of the tools that has a place for certain things at least in the way I do them currently :)
In fact, I had to interfere a bit to make my Zombies make bad decisions as to NOT always follow you, so I don't even apply it as specified at the moment, hahahahahaha. Also, because I want my Zombies to "swarm" the Hero if they reach him, I break out of this algo and use something different altogether at very close range.
[edit] btw, I get pretty bad headaches in a lot of 3D games too. Usually the culprit for me is motion blur. I always turn that off now.
This article provides a very good explanation of A* and seems to be linked in most places where I've seen people ask for A* tutorials
These sweet gifs show the difference in node visitation:
Dijkstra's
A*