Transforming several objects via OnGUI in Unity
Howzit dudes, so I finally got cracking with Unity and need some advice. I am trying to replicate the puzzle game Cryptica I've been playing on my Android where you have to match cubes with their corresponding patterns on a puzzle board. In Cryptica all cubes move simultaneously and their relative positions can only be altered when hitting the end of the board or when blocked by static cubes.
Here is my [Updated] code:
If I attach the script to each cube then OnGUI will only move one cube at a time. How do I transform several objects simultaneously? Is there a better way to approach this?
Shot!
Here is my [Updated] code:
var cubeMovement = 11; var greenCube; function Start() { greenCube = GameObject.Find("Green"); } function Update () { } function OnGUI () { //Move dynamic cubes if (GUI.Button (Rect (250,150,50,50), "Up")) { moveUp(); } if (GUI.Button (Rect (350,250,50,50), "Right")) { moveRight(); } if (GUI.Button (Rect (250,350,50,50), "Down")) { moveDown(); } if (GUI.Button (Rect (150,250,50,50), "Left")) { moveLeft(); } } function moveUp() { transform.Translate(cubeMovement,0,0); greenCube.transform.Translate(cubeMovement,0,0); } function moveRight() { transform.Translate(0,0,-cubeMovement); greenCube.transform.Translate(0,0,-cubeMovement); } function moveDown() { transform.Translate(-cubeMovement,0,0); greenCube.transform.Translate(-cubeMovement,0,0); } function moveLeft() { transform.Translate(0,0,cubeMovement); greenCube.transform.Translate(0,0,cubeMovement); }
If I attach the script to each cube then OnGUI will only move one cube at a time. How do I transform several objects simultaneously? Is there a better way to approach this?
Shot!
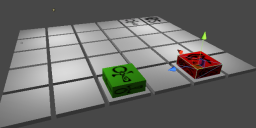
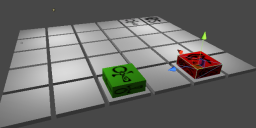
Screen Shot 2012-07-09 at 3.48.22 PM.png
742 x 330 - 71K
Comments
Also, I put your code into code tags.
(minus the space in the right brace)
Try building a controller object that maintains a list of all the cubes and moves each of them as necessary. The controller would be the only object with any GUI calls :)
You can have a list of GameObjects that you go through and call the procedure from the control object with. (So the control object has a list of GameObject and the OnGUI)
So the invisible control object would have a script something like this (C#-like pseudocode):
And the script in the cubes would be something like:
I hope that makes more sense? The control object will be separate and just call the function on the game objects that require the movement.